Enrich traces with Execution Tags
Execution Tags allow you to dynamically add dimensions to invocations and requests so that they can be identified, searched for, and filtered in Lumigo, helping you simplify the complexity of monitoring distributed applications.
Skip to the Quick Bytes video for Execution Tags
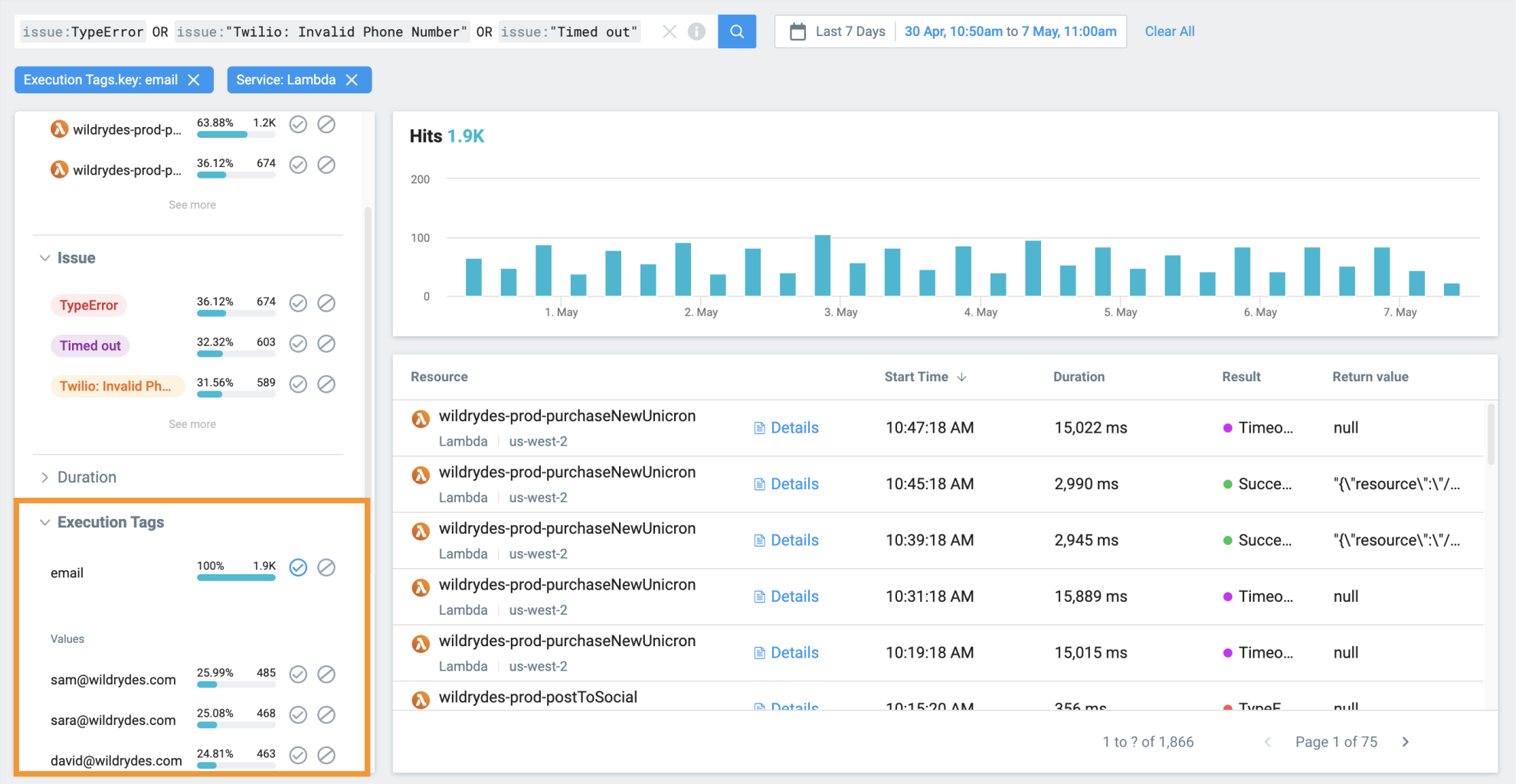
You an add Execution Tags to
- Execution Tags in AWS Lambda functions.
- Execution Tags in OpenTelemetry and Containers.
Execution Tags in Lambda functions
From the command line, install the lumigo tracer
pip install lumigo_tracer
npm install @lumigo/tracer --save
using Amazon.Lambda.Core;
using Lumigo.DotNET;
using Lumigo.DotNET.Instrumentation;
using Lumigo.DotNET.Utilities.Extensions;
using System.Net.Http;
[assembly: LambdaSerializer(typeof(Amazon.Lambda.Serialization.Json.JsonSerializer))]
namespace HelloDotNet6 {
public class Function : LumigoRequestHandler
{
public Function()
{
LumigoBootstrap.Bootstrap();
}
public async Task<string> Handler(string input, ILambdaContext context)
{
return await Handle(input, context, async () =>
{
return "\"Hello world\"";
}
);
}
}
}
Adding for Auto-Traced functions
Add an execution tag to your handler’s code
from lumigo_tracer import add_execution_tag
add_execution_tag(“user_id”,user_id)
const lumigo = require('@lumigo/tracer');
lumigo.addExecutionTag('<key>', '<value>');
Adding for Manual traced functions
from lumigo_tracer import lumigo_tracer
from lumigo_tracer import add_execution_tag
@lumigo_tracer
def my_lambda(event, context):
print('I can finally troubleshoot!')
add_execution_tag(“user_id”,user_id)
const lumigo = require('@lumigo/tracer');
lumigo.addExecutionTag('<key>', '<value>');
public async Task<string> Handler(string input, ILambdaContext context)
{
return await Handle(input, context, async () =>
{
this.AddExecutionTag("<key>", "<value>");
return "\"Hello world\"";
}
);
}
To find out more about Manual Tracing visit AWS Lambda Manual Tracing
Auto execution tags from the Lambda event
Capability supported in Lambda Python and Node.js
Automatically adding execution tags from the Lambda event is supported in AWS Lambda using Node.js and Python runtimes.
To configure execution tags that will be driven directly from the event, add an environment variable to the Lambda function with the name of the relevant key. We support only the python and node runtimes.
For example, to configure an auto tag from the key product_id, add the environment variable:
Key | Value |
---|---|
LUMIGO_AUTO_TAG | product_id |
To configure multiple auto tags, such as product_id
and source
, add:
Key | Value |
---|---|
LUMIGO_AUTO_TAG | product_id,source |
For a nested field, such as name
inside the value of the key source
, the syntax is:
Key | Value |
---|---|
LUMIGO_AUTO_TAG | source.name |
Execution Tags in OpenTelemetry and Containers
Execution tags in the container tracers like the Lumigo OpenTelemetry Distros for Python and JS are created using the OpenTelemetry Span.setAttribute()
API.
For example, using the Lumigo OpenTelemetry Distros JS with Typescript, this code will add the foo
execution tag with value bar
:
// Typescript
import { trace } from '@opentelemetry/api';
/*
* In Node.js 14+, the '?' coalescing operator ensures that your code is
* safe even if the tracing is not active, and `trace.getActiveSpan()` returns
* `undefined`.
*/
trace.getActiveSpan()?.setAttribute('lumigo.execution_tags.foo','bar');
For more information on how to set execution tags with the Lumigo OpenTelemetry Distros, refer to the Lumigo OpenTelemetry Distro for JS and Lumigo OpenTelemetry Distro for Python documentation pages.
For other OpenTelemetry SDKs, the implementation of Span.setAttribute
API will work, as long as you add lumigo.execution_tags.
as prefix to the attribute name, and do not use other .
characters in the name.
Execution Tags naming limits and requirements
- Execution tags can be added only to a traced function or container.
- The maximum number of executions tags per invocation is 50.
- Key length must be between 1 and 50.
- Value length must be between 1 and 70.
Lumigo Quick Bytes - Execution Tags
Learn More
Execution Tags based Alerts
Learn how to create Event Alert and Metric Alert alerts at the invocations and request level.
Explore Execution Tags
Learn how to filter by Execution Tags to easily find specific invocations and requests on the Explore.
Create Custom Widgets
Learn how to create customized trace-based metrics to get accurate insights.
Updated 5 months ago